
{{text-cta}}
Customer expectations are skyrocketing—from cheap shipping prices to fast text notifications to 24/7 customer service access and more. So, achieving those needs necessitates meticulous coordination of all systems within a firm. But how can you keep everything structured and simplified if your CSM software isn't built to interact with your big data platform or the tools you use for digital marketing campaigns?
This is where middleware enters the picture.
Middleware is software that connects other applications, tools, and databases to provide users with unified services. It's often referred to as the "glue" that binds together various software systems and devices.
Web servers, content management systems, application servers, and other technologies that support application design and delivery are all examples of middleware.
The importance of middleware has been emphasized by the use of network applications by tech firms. SaaS organizations create enterprise-wide information systems by employing middleware to combine self-sustaining applications with new software updates.
How middleware works
Middleware is a section of software that connects operating systems and communication protocols. It does the following:
- Conceal a fractured and dispersed network.
- From a diverse set of software applications, create homogeneity.
- Provide a consistent user interface for developers to aid in application development, usability, and interoperability.
- Provide a set of general-purpose services that allow applications to communicate with one another and prevent systems from repeating tasks.
Middleware also aids application development by providing standard programming abstractions, covering application heterogeneity and the spread of underlying hardware and operating systems, and concealing low-level programming specifics.
While all middleware provides communication duties, the type of middleware a firm chooses will be determined by the service being used and the sort of data that has to be conveyed. This can involve transaction management, security authentication, email databases, application servers, directories, and web servers.
Why choose middleware?
The goal of middleware is to facilitate communication between different components of an application or even between separate apps. Businesses might expect to benefit from middleware in the following ways:
- More brief transition periods.
- Innovative solutions.
- Easily accessible workplace tools.
- Efficient connectivity.
The Android operating system, for example, uses middleware to run its software more quickly, efficiently, and with a better user experience. Android not only runs on the Linux kernel and has an application architecture, but it also has a middleware layer that includes libraries that provide services like:
- Screen display.
- Web browsing.
- Multimedia.
- Data storage.
All this, in turn, helps the page(s) you intend to create. Customers can be drawn to your leading site and promotional landing pages, where they can be converted into users. It's referred to as user acquisition. It's critical to understand that you collect massive data when you drive traffic to your website.
Middleware and its applications
- Injecting Data into Requests.
Middleware can inject extra data into a request that the application can then use. Take Django's Authentication Middleware, for example, which adds a user object to every legitimate appeal. This is a simple way for the view and other middleware to get information about the currently logged-in user by calling requests. Of course, there will always be new developments, and tech-related podcasts can help you stay on top of those updates, which is one of many benefits of podcasts.
- Filtering Requests.
Middleware can be designed to filter out incorrect or potentially dangerous requests and return them promptly (typically with an error response), effectively stopping them from continuing. One artificial example is the CSRF middleware mentioned earlier, which blocks out requests received from various domains.
Another example could be RBAC (Role-Based Access Control) middleware, which stops users from reading or editing resources they are not authorized. Another example is geo-blocking middleware, which blocks requests from specific geographic areas.
- Performing Analytics, Logging, and Other Miscellaneous Tasks.
Some middleware makes use of the information contained inside the request/response rather than altering it directly. To put it another way, this is read-only' middleware. This is quite common in all the leading eCommerce platforms. For example, consider an analytics middleware that keeps the details of all requests entering the system in a database, including the associated user, URL, timestamp, and so on.
After that, the data would be analyzed to find helpful information or trends. Another example is a usage monitoring middleware that keeps track of how much of a user's use quota they've used up.
Middleware in Django
In Django, middleware is a small plugin that runs in the background while processing requests and responses. The application's middleware is utilized to complete a task. Security, session, CSRF protection, and authentication are examples of functions.
Django comes with a variety of built-in middleware and also enables us to develop our own. For example, see the Django project's settings.py file, which contains various middleware used to offer functionality to the application. Security Middleware, for example, is used to keep the application secure.
// settings.py

{{text-cta}}
Types of middleware
In Django, there are two types of middleware:
- Built-in Middleware.
- Custom Middleware.
When you start a Django project, it comes with built-in middleware by default. The default Middleware can be found in your project's settings.py file.
Custom Middleware: you can create your middleware to utilize across your entire project.
Creating your middleware (Custom Middleware)
A callable that exerts a get response callable and generates a middleware is known as a middleware factory. A middleware, like a view, is a callable that takes a request and delivers a response. If you are wondering how to make a prototype, follow the below steps.
A middleware can be written as the following function:
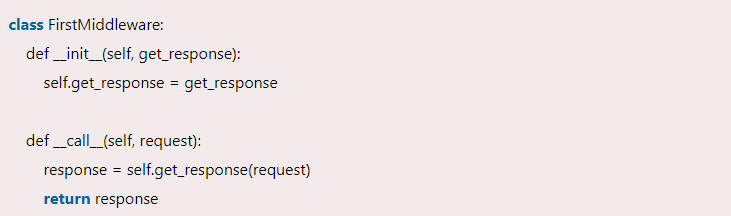
If this is the last stated middleware, the get_response callable provided by Django could be the actual view, or it could be the next middleware in the chain. The current middleware doesn't need to know or care what it is; it only needs to know that it represents whatever follows.
The get_response callable for the last middleware in the chain will be a wrapper function from the handler that applies view middleware, calls the view with reasonable URL arguments and uses template-response and exception middleware.
Middleware can handle synchronous Python exclusively (the default), asynchronous Python only, or both. See Asynchronous support for further information on advertising what you support and identify the type of request you're getting.
Middleware can be found in any Python path.
__init__(get_response)
Middleware factories need to allow a get_response argument. For the middleware, you may also set up some global states. There are a few things to keep in mind:
Because Django only uses the get response argument to initialize your middleware, you can't have __init__() require any additional arguments.
In contrast to the __call__() method, which is called once every request, __init__() is only called once the Web server is started.
Other Middleware Hooks
process_view
When Django receives a request and directs it to a view, this function is invoked. What makes it unique from the __call__ method? We now have access to the view function to which Django is routing the request and any additional arguments (args and kwargs) that may be supplied to it. Because path parameters and respective values are typically supplied as kwargs, our middleware must implement the process_view method if it needs access to them.
The method must return either None, in which case Django will proceed processing the response as usual, or a HttpResponse, in which instance it will return with that result right away.
process_exception
When a view throws an exception that isn't caught within the view, this function is called. As a result, a process_exception is called when the request has gotten and been returned from the view.
The process_exception method, like the others, must return either None or a HttpResponse.
Process_template_response
This function is also called once the view has completed its execution. It's only called if the answer has a render () method, indicating that a template is rendered. If necessary, you can use this method to change the template's content, including its context data. Reach out to the online community for further clarifications.
Middleware Registration
Once you've developed your middleware class, you'll need to register it with your Django project so that it can be added to the middleware sequence that each request goes through. Then, add it to the MIDDLEWARE list in your main settings.py file to complete.

The full path of your middleware class, in string form, should be included in the entry.
It's worth noting that the order in which middleware appears in this list is crucial. This is because some middleware may rely on another to work well or obstruct another's ability to function correctly. Most security-related middleware is near the top of the list, where it can notice and filter out potentially hazardous requests early. Most custom middleware is added at the end of the list as a rule of thumb. However, this isn't always the case, especially if your middleware is security-related, as mentioned above. On a case-by-case basis, you must select where to put your middleware entry.
It is common to struggle when going through such a process to begin with. However, reaching out to a professional can get this work done in no time.
Asynchronous support
Any combination of synchronous and asynchronous requests can be dealt with via middleware. If Django cannot support both, it will adjust requests to match the middleware's requirements, albeit at a performance cost.
Django assumes that your middleware can only handle synchronous requests by default. Set the subsequent attributes on your middleware factory function or class to change these assumptions:
The boolean sync capability indicates if the middleware can support synchronous requests—defaults to True.
The boolean async capability indicates if the middleware can handle asynchronous requests—defaults to False.
If both sync capable and async capable = True in your middleware, Django will pass the request to it without converting it. In this situation, you may verify if the get response object you're given is a coroutine function using asyncio.iscoroutinefunction() to see if your middleware will accept async requests.
The sync or async nature of the get response method must be reflected in the returned callable. For example, you must return a coroutine function if you have an asynchronous get_response (async def).
The sync or async nature of the get response method must be reflected in the returned callable. You must return a coroutine function if you have an asynchronous get_response (async def).
If given, the process_view, process_template response, and process_exception functions should also be adjusted to match the sync/async mode. If you don't, Django will individually adjust them as needed, resulting in a performance penalty.
Here's an example of a middleware function that can handle both:

Please Note: The type of call you get may not match the underlying view if you specify a hybrid middleware that supports synchronous and asynchronous calls. This is because the middleware call stack in Django will be optimized to have as few sync/async transitions as possible.
If there is additional, synchronous middleware between you and the view, you may be called in sync mode even if you are encapsulating an async view.
Things to remember when using middleware:
- A middleware only need to extend from the class object
- Order of middleware is essential.
- A middleware can choose to implement some of the methods while ignoring others.
- process_request may be implemented by a middleware; however, process_response and process_view may not.
Users can use middleware to make requests like submitting forms in a web browser or enabling the web server to deliver dynamic web pages depending on a user's profile. It also plays a part in mobile app marketing since middleware allows distributed applications to communicate and manage data by acting as a covert translation layer.
Wrapping up
If your business is heavily reliant on data, you should consider adding middleware to help you combine data from numerous apps and systems. Integration makes data flow between applications much more accessible and helps your organization focus on other critical parts of its business because manual processes are no longer required.
The corporate environment has become more dynamic, necessitating the integration of formerly independent applications and the development of enterprise-wide information systems.
Furthermore, in today's highly competitive economic world, middleware enables companies to develop and market new products much more quickly. When selecting a middleware solution, consider your organization's needs and the type of infrastructure it employs.
Learn from Nana, AWS Hero & CNCF Ambassador, how to enforce K8s best practices with Datree
Headingajsdajk jkahskjafhkasj khfsakjhf
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Suspendisse varius enim in eros elementum tristique. Duis cursus, mi quis viverra ornare, eros dolor interdum nulla, ut commodo diam libero vitae erat. Aenean faucibus nibh et justo cursus id rutrum lorem imperdiet. Nunc ut sem vitae risus tristique posuere.